When Laravel E-commerce App Meets AI: Unleashing the Power of SharpAPI in 10 Use Cases
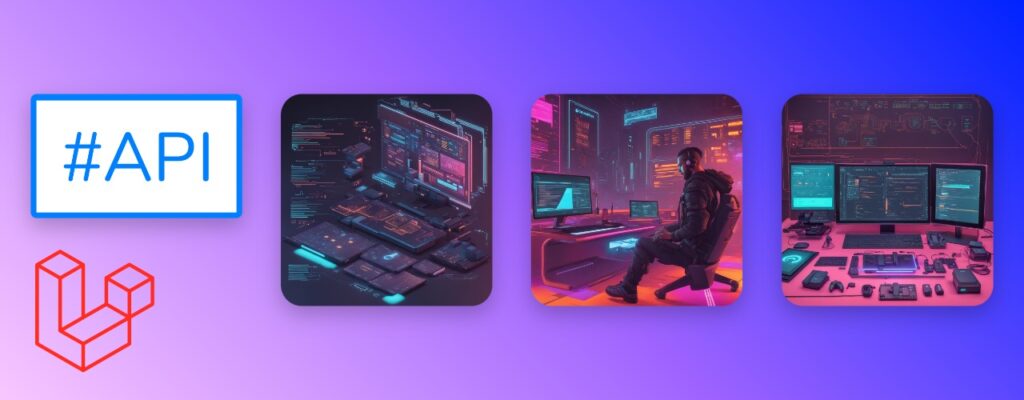
Introduction
Hey fellow Laravel enthusiasts! In the ever-evolving landscape of e-commerce, integrating AI into your Laravel applications is not just a luxury, it’s a necessity. With SharpAPI’s Laravel package, it’s now easier than ever. In this blog, I’ll guide you through 10 transformative use cases for enhancing your Laravel-based e-commerce applications using SharpAPI.
Please refer to the package GitHub page for full documentation.
It can save countless hours and supercharges any app with AI capabilities with just a couple of lines of code.
Let’s dive in!
Installation
First things first, let’s get the SharpAPI Laravel package installed. It’s a breeze:
composer require sharpapi/sharpapi-laravel-client
Next, grab your API key by registering at SharpApi.com, and set it in your .env
:
SHARP_API_KEY=your_api_key_here
That’s it! You’re all set to supercharge your app.
Use Cases and Code Samples
1. Generating Product Descriptions
Create engaging product descriptions in seconds.
Provide as many details and parameters of the product to get the best marketing introduction possible. Comes in handy with populating product catalogue data and bulk product processing. Here’s how:
$statusUrl = \SharpApiService::generateProductIntro('Razer Blade 16 Gaming Laptop: NVIDIA GeForce RTX 4090-13th Gen Intel 24-Core i9 HX CPU - 16 inch Dual Mode Mini LED (4K UHD+ 120Hz & FHD+ 240Hz) - 32GB RAM - 2TB SSD - Compact GaN Charger - Windows 11 NVIDIA GEFORCE RTX 4090 GRAPHICS,13TH GEN INTEL CORE I9 13950HX PROCESSOR', 'English');
$result = \SharpApiService::fetchResults($statusUrl);
dd($result->getResultJson());
Example Response:
{
"product_intro": "Experience the ultimate gaming and content creation powerhouse with the Razer Blade 16 Gaming Laptop. Featuring the NVIDIA GeForce RTX 4090 graphics and the 13th Gen Intel Core i9 HX CPU, this laptop delivers unparalleled performance for both gaming and resource-intensive tasks. The next-gen dual-mode Mini LED display offers stunning 4K resolution for creative work and ultra-fast refresh rates for smooth gameplay. With a compact GaN charger and an anodized aluminum unibody, this laptop is designed for portability without compromising on power and durability."
}
2. Product Review Sentiment Analysis
Understand your customers better by analyzing sentiments in their reviews.
Parses the customer’s product review and provides its sentiment (POSITIVE/NEGATIVE/NEUTRAL) with a score between 0-100%. Great for sentiment report processing for any online store:
$statusUrl = \SharpApiService::productReviewSentiment('This console has amazing graphics!');
$result = \SharpApiService::fetchResults($statusUrl);
dd($result->getResultJson());
Example Response:
{
"opinion": "POSITIVE",
"score": 94
}
3. Category Suggestions for Products
Automatically categorize products to enhance user navigation.
Generates a list of suitable categories for the product with relevance weights as a float value (1.0-10.0) where 10 equals 100%, the highest relevance score. Provide the product name and its parameters to get the best category matches possible. Comes in handy with populating product catalogue data via forms and bulk products processing.
$statusUrl = \SharpApiService::productCategories('Razer Blade 16 Gaming Laptop: NVIDIA GeForce RTX 4090-13th Gen Intel 24-Core i9 HX CPU - 16 inch Dual Mode Mini LED (4K UHD+ 120Hz & FHD+ 240Hz) - 32GB RAM - 2TB SSD - Compact GaN Charger - Windows 11', 'English');
$result = \SharpApiService::fetchResults($statusUrl);
dd($result->getResultJson());
Example Response:
[
{
"name": "Gaming Laptops",
"weight": 10
},
{
"name": "Computer Hardware",
"weight": 9
},
{
"name": "Electronics",
"weight": 8
},
{
"name": "Technology",
"weight": 7
}
]
4. Personalized Thank You Emails
Automate personalized thank-you emails post-purchase.
The response content does not contain the title, greeting or sender info at the end so you can personalize the rest of the email more easily.
$statusUrl = \SharpApiService::generateThankYouEmail('"[I'M From] Honey Mask 4.23oz | wash off type, real honey 38.7%, Deep moisturization, Nourishment,Hydrating and Clear Complexion.', 'English');
$result = \SharpApiService::fetchResults($statusUrl);
dd($result->getResultJson());
Example Response:
{
"email": "We would like to sincerely thank you for purchasing the [I'M From] Honey Mask 4.23oz | wash off type, real honey 38.7%, Deep moisturization, Nourishment, Hydrating and Clear Complexion. We appreciate your support and hope that our product will provide your skin with the nourishment and hydration it needs. If you have any questions or need further assistance, please feel free to contact us. Your satisfaction is our priority, and we are here to ensure that you have a positive experience with our products. Thank you once again for choosing our products. We value your trust and look forward to serving you again in the future."
}
5. Content Translation
Reach a global audience by translating product descriptions.
You can also use this endpoint to translate any part of your marketing automation workflow or communication with your customers. API supports 80 languages at the moment.
$statusUrl = \SharpApiService::translate('This phone has the best camera on the market.', 'Spanish');
$result = \SharpApiService::fetchResults($statusUrl);
dd($result->getResultJson());
Example Response:
{
"content": "Este teléfono tiene la mejor cámara del mercado.",
"from_language": "English",
"to_language": "Spanish"
}
6. Spam Content Detection
Keep your product reviews clean and relevant. You can process any kind of user input via this method (like comments etc).
Provides a percentage confidence score and an explanation for whether it is considered spam or not. This information is useful for moderators to make a final decision.
$statusUrl = \SharpApiService::detectSpam('Hello, this is John from Finance Plus. I've called before, We've helped other individuals like you improve their credit. Please give me a call later.');
$result = \SharpApiService::fetchResults($statusUrl);
dd($result->getResultJson());
Example Response:
{
"pass": false,
"score": 80,
"reason": "The provided content is likely spam. It exhibits characteristics common in spam messages, including unsolicited outreach, a generic and impersonal tone, and a call to action for credit improvement without specific details about the individual situation. The mention of having called before may be an attempt to create a false sense of familiarity. The overall content raises suspicion of potential spam."
}
7. Extracting Contact Information: Phones
Extract phone numbers and emails from customer inquiries efficiently.
Example below parses the provided text for any phone numbers and returns the original detected version and its E.164 format. Might come in handy in the case of processing and validating big chunks of data against phone numbers or f.e. if you want to detect phone numbers or emails in places where they’re not supposed to be.
$statusUrl = \SharpApiService::detectPhones('Where to find us? Call with a sales tech advisor: Call: 1800-394-7486 or our Singapore office +65 8888 8888');
$result = \SharpApiService::fetchResults($statusUrl);
dd($result->getResultJson());
Example Response:
[
{
"parsed_number": "+18003947486",
"detected_number": "1800-394-7486"
},
{
"parsed_number": "+6588888888",
"detected_number": "+65 8888 8888"
}
]
8. Extracting Contact Information: Emails
Parses the provided text for any possible emails. Might come in handy in case of processing and validating big chunks of data against email addresses or if you want to check if in places where they’re not supposed to be or might be passed in a weird format (like example below: Hidden_email[at]amazon.com
)
$statusUrl = \SharpApiService::detectEmails('Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum. Contact us at example@email.com or lorem.ipsum@email.com for more information. Hidden_email[at]amazon.com');
$result = \SharpApiService::fetchResults($statusUrl);
dd($result->getResultJson());
Example Response:
[
"example@email.com",
"lorem.ipsum@email.com",
"Hidden_email@amazon.com"
]
9. Summarizing Text for Quick Insights
Quickly summarize long product details or reviews for easier consumption.
Just dump all the information and parameters about the product provided by the vendor for the best results. Perfect for generating marketing introductions of longer texts:
$statusUrl = \SharpApiService::summarizeText('Xbox Wireless Controller - Carbon Black Includes Xbox Wireless and Bluetooth technology for wireless gaming on supported consoles, Windows 10 PCs, Android phones, and tablets. Stay on target with textured grip on the triggers, bumpers, and back case. A new hybrid D-pad for accurate, yet familiar input.
Make the controller your own by customizing button mapping with the Xbox Accessories app. Manufacturer Microsoft Item model number QAT-00003 Product Dimensions 17.8 x 17.7 x 7.2 cm; 455 Grams ASIN B08HNSLYYD', 'English');
$result = \SharpApiService::fetchResults($statusUrl);
dd($result->getResultJson());
Example Response:
{
"summary": "Experience gaming like never before with the Xbox Wireless Controller in Carbon Black. This sleek controller offers the freedom of wireless gaming on supported consoles, Windows 10 PCs, Android phones, and tablets, giving you the flexibility to play wherever you go. Its textured grip ensures you stay on target during intense gameplay, while the new hybrid D-pad provides accurate and familiar input. Plus, you can personalize your gaming experience by customizing button mapping using the Xbox Accessories app. Elevate your gaming with this high-quality controller from Microsoft, designed for precision and comfort."
}
10. SEO Tag Generation for Products
Automatically generate META tags for product pages to boost SEO.
Make sure to include links to the website and pictures URL to get as many tags populated as possible.
$statusUrl = \SharpApiService::generateSeoTags('Spigen Urban Fit Designed for New MacBook Pro 14 Inch and 16 Inch Models (2023 and 2021) - Protective Hard Shell Case - Black, Brand Spigen, Color Black, Compatible Devices MacBook Pro 14 inch, Form Factor Case, Shell Type Hard, About this item, Sleek and minimal design with a premium knit fabric, Durable but slim enhanced daily protection, Precise cutouts around ports for easy access, Overheating prevention with elevated bottom, Designed specifically for your MacBook Pro 14 inch with new M3 / M3 Pro / M3 Max and M2 Pro / M2 Max / M1 Pro / M1 Max Chip (A2779 / A2442 / 2023 / 2021), URL: https://www.amazon.com/Spigen-Urban-Designed-MacBook-Shell/dp/B09R2KCXD2?ref_=ast_sto_dp&th=1 , product photo: https://m.media-amazon.com/images/I/71WagddZ64L._AC_SX679_.jpg', 'English');
$result = \SharpApiService::fetchResults($statusUrl);
dd($result->getResultJson());
Example Response:
{
"meta_tags": {
"title": "Spigen Urban Fit Designed for MacBook Pro 14 Inch and 16 Inch Models",
"description": "Sleek and minimal design with a premium knit fabric. Durable but slim enhanced daily protection. Precise cutouts around ports for easy access. Overheating prevention with elevated bottom.",
"keywords": "Spigen, Urban Fit, MacBook Pro 14 inch, protective case, hard shell case, black, M3, M3 Pro, M3 Max, M2 Pro, M2 Max, M1 Pro, M1 Max",
"author": "",
"og:url": "https://www.amazon.com/Spigen-Urban-Designed-MacBook-Shell/dp/B09R2KCXD2?ref_=ast_sto_dp&th=1",
"og:type": "",
"og:image": "https://m.media-amazon.com/images/I/71WagddZ64L._AC_SX679_.jpg",
"og:title": "Spigen Urban Fit Designed for MacBook Pro 14 Inch and 16 Inch Models",
"og:site_name": "",
"og:description": "Sleek and minimal design with a premium knit fabric. Durable but slim enhanced daily protection. Precise cutouts around ports for easy access. Overheating prevention with elevated bottom.",
"twitter:card": "summary_large_image",
"twitter:image": "https://m.media-amazon.com/images/I/71WagddZ64L._AC_SX679_.jpg",
"twitter:title": "Spigen Urban Fit Designed for MacBook Pro 14 Inch and 16 Inch Models",
"twitter:description": "Sleek and minimal design with a premium knit fabric. Durable but slim enhanced daily protection. Precise cutouts around ports for easy access. Overheating prevention with elevated bottom.",
"twitter:creator": ""
}
}
Conclusion
There you have it, Laravel devs! With these 10 AI-powered enhancements via SharpAPI, your e-commerce app will not only provide a better user experience but also streamline your operations. It’s time to let AI do the heavy lifting, so you can focus on innovating and growing your business. Happy coding!